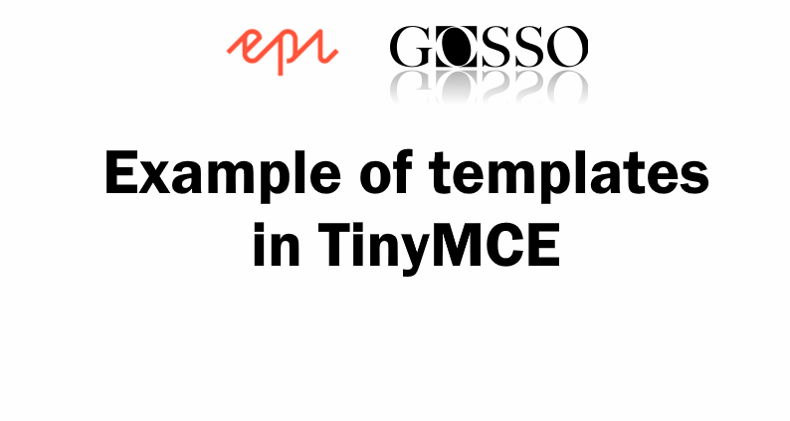
#TinyMCE Template plugin example with “insert-after” functionality
Example of use of templates, and with a feature trick to insert in bottom of the editor. #TinyMCE
Published 1st of October 2018
TinyMCE version 4 and later…
The Template plugin is easy to implement (check further down and documentation), but by default the template is inserted where the marker is. And I wanted the template to be added at the end of the document.
I looked into adding a new “insert after”-button on template-plugin UI, but that wasn’t doable.
Solution
Tag the html-templates and use the BeforeSetContent-event to hi-jack the pipe with your logic.
So, in the HTML templates I did start with a data attribute “<div data-insertafter” meaning it should have that behavior.
Template example:
<div data-insertafter class="tmpl1"> <div class="padding"> <h2>Template 1</h2> <p>Fusce accumsan ligula at dolor vulputate malesuada.</p> </div> </div>
- Implement the standard template plugin
- Register a custom plugin in tinymce.PluginManager
"use strict"; var tinymce = tinymce || {}; tinymce.PluginManager.add('insertaftertemplate', function (editor, url) { editor.on('BeforeSetContent', function (e) { if (e.content.include("<div data-insertafter")) { editor.setContent(editor.getContent() + '<div class="row"></div>'); editor.selection.select(editor.getBody(), true); editor.selection.collapse(false); e.content = e.content.replace("data-insertafter", ""); } }); return { getMetadata: function () { return { name: 'Insert after for Templates', url : 'https://devblog.gosso.se/?p=847' }; } }; });
How to implement Template Plugin
All you need is …
1. add the source code to page
<script src=”/tinymce/js/tinymce.min.js”></script> <script src=”/ClientResources/Scripts/tinymce/plugins/template_insert_hack/editor_plugin_v4.js “></script>
2. configure the template plugin
tinymce.init({ selector: "textarea", // change this value according to your HTML plugins: "template insertaftertemplate", // menubar: "insert", toolbar: "template", templates: "/dir/.../templates.htm" //json file });
I choose to configure the templates in external file with json format “template.htm”
[ {"title": "Template 1", "description": "inserting inline", "url": "/ClientResources/Scripts/tinymce/plugins/template_insert_hack/tmpl1.htm"}, {"title": "Template 1 (after)", "description": "Inserting after", "url": "/ClientResources/Scripts/tinymce/plugins/template_insert_hack/tmpl1a.htm"}, {"title": "Template 2", "description": "inserting inline", "url": "/ClientResources/Scripts/tinymce/plugins/template_insert_hack/tmpl2.htm"}, {"title": "Template 2 (after)", "description": "Inserting after", "url": "/ClientResources/Scripts/tinymce/plugins/template_insert_hack/tmpl2a.htm"} ]
Example filestructure
Register in a custom plugin in Episerver
With package Episerver.Cms.Tinymce v2 and later, you use TinyMceInitialization like this:
using EPiServer.Cms.TinyMce.Core; using EPiServer.Framework; using EPiServer.Framework.Initialization; using EPiServer.ServiceLocation; namespace Gosso.Mvc.Business.Initialization { [ModuleDependency(typeof(TinyMceInitialization))] public class CustomizedTinyMceInitialization : IConfigurableModule { public void Initialize(InitializationEngine context) { } public void Uninitialize(InitializationEngine context) { } public void ConfigureContainer(ServiceConfigurationContext context) { context.Services.Configure<TinyMceConfiguration>(config => { config.Default() .AddSetting("template_popup_width", 1100) .AddSetting("templates", "/ClientResources/Scripts/tinymce/plugins/template_insert_hack/templates.htm") .AddExternalPlugin("insertaftertemplate", "/ClientResources/Scripts/tinymce/plugins/template_insert_hack/editor_plugin_v4.js"); }); } } }
Resources
SEO-Terms
- Insert before, insert bottom templates plugin
- Insert data after the content template plugin
- tinymce 4 template plugin example
- using tinymce template
About the author
Luc Gosso
– Independent Senior Web Developer
working with Azure and Episerver
Twitter: @LucGosso
LinkedIn: linkedin.com/in/luc-gosso/
Github: github.com/lucgosso