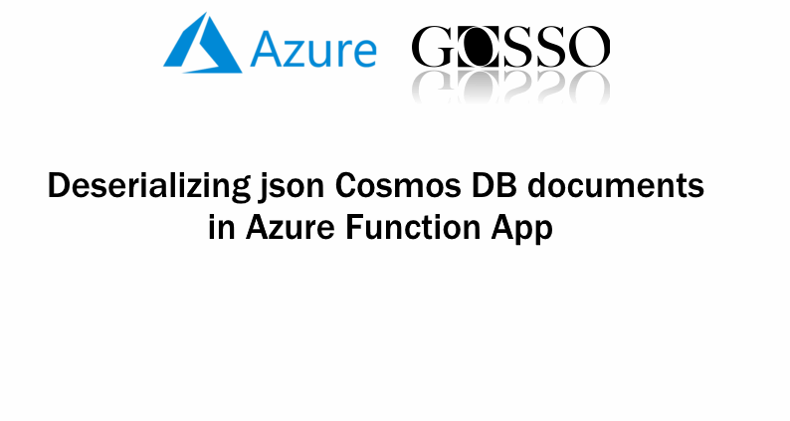
Deserializing json Cosmos DB objects in Azure Function App
Simple Note to self how to deserialize json data from Cosmos DB to custom object with Newtonsoft.Json.JsonConvert.DeserializeObject<>
Example function app with Cosmos DB SQL API
Example 1: Getting data from Cosmos DB with SQL API then deserializing the data to custom object.
JsonConvert.DeserializeObject<T>(json-string)
using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Http; using Microsoft.Azure.WebJobs; using Microsoft.Azure.WebJobs.Extensions.Http; using Microsoft.Azure.WebJobs.Host; using Newtonsoft.Json; namespace EpiWorldFunctions.CertifiedDevs { public static class GetDevs { [FunctionName("GetDevs")] public static HttpResponseMessage Run([HttpTrigger(AuthorizationLevel.Anonymous, "get", Route = "GetDevs/{type}/{company}")]HttpRequestMessage req, string company, string type, [DocumentDB(Manager.DatabaseName, "devs", ConnectionStringSetting = Manager.DbConnString, SqlQuery = "SELECT top 1 * FROM c where c.type={type} and c.count>0 order by c._ts desc")] IEnumerable<object> documents , TraceWriter log) { Manager.DbDocument deserializedDbDocument = JsonConvert.DeserializeObject<Manager.DbDocument>(documents.FirstOrDefault()?.ToString()); if (company == "Unknown") deserializedDbDocument.Devs = deserializedDbDocument.Devs.Where(item => item.Company=="") .Select(x => x) .ToList(); else if (company!="everyone") deserializedDbDocument.Devs = deserializedDbDocument.Devs.Where(item => System.Net.WebUtility.HtmlDecode(item.Company).Contains(company)) .Select(x=>x) .ToList(); string message = $"type: {type} | company: {company} | returns: {deserializedDbDocument.Devs.Count}"; log.Info(message); System.Diagnostics.Trace.WriteLine(message); return req.CreateResponse(HttpStatusCode.OK, deserializedDbDocument, "application/json"); } } }
Business logic (Manager) and structs:
using Microsoft.Azure.WebJobs.Host; using System; using System.Collections.Generic; using System.Linq; using System.Net; using Newtonsoft.Json; using Newtonsoft.Json.Serialization; namespace EpiWorldFunctions { public class Manager { public const string DbConnString = "AzureWebJobsDocumentDBConnectionString"; //this file is set in local.settings.json public const string DatabaseName = "databasename-not-collection"; public struct DbDocument { [JsonProperty("date")] public DateTime Date; [JsonProperty("devs")] public List<Developer> Devs; [JsonProperty("count")] public int Count; [JsonProperty("type")] public string Type; } public struct Developer { [JsonProperty("name")] public string Name; [JsonProperty("company")] public string Company; [JsonProperty("url")] public string Url; } } }
Further reading
- Http Trigger #Azure functions with CosmosDB SQL API
- https://www.newtonsoft.com/json/help/html/DeserializeObject.htm
About the author
Luc Gosso
– Independent Senior Web Developer
working with Azure and Episerver
Twitter: @LucGosso
LinkedIn: linkedin.com/in/luc-gosso/
Github: github.com/lucgosso