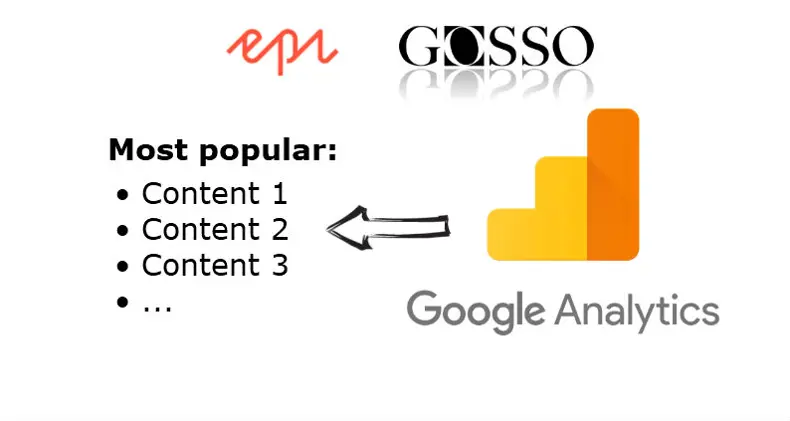
Most popular using Google Analytics API
Full tutorial how to use your Google analytics data to list content on your site order by page views.
Published 1st of July 2018
Google.Apis.AnalyticsReporting.v4 or v3
Wouldn’t it be cool to have an Linq extension method .OrderByMostPopular()?
In this blog post I will show you how you can implement a most popular trending content listing on your site with help of Google Analytics data. I will use my favorite CMS Episerver in my example but same approach can be used in any C# CMS.
The steps in summary
-
- Start register the page id and type using custom dimensions
- Enable the API in Google APIs and Services
- Register a service account
- Download a service key file
- Register the service account in Google Analytics User management
- Download nuget Google.Apis.AnalyticsReporting.v4
- Configure your site
- Use my class GoogleAnalyticsService or Linq extension “OrderByMostPopular“
Register the page id and type using custom dimensions
In google analytics, find your way to your account by clicking “admin” and “property”, click Custom definitions/custom dimensions.
Create two custom dimensions one for page id or page guid, one type id, name them anything that mathers for you. (type id is for you to be able to filter by type, minimizing the workload)
Send the data on page view
When sending to GA, the names are always “dimension1” and “dimension2” in the order you added the custom dimension.
ga('create', 'UA-55555-01', 'none'); ga('set', 'dimension1', '@Model.CurrentPage.ContentGuid'); ga('set', 'dimension2', '@Model.CurrentPage.ContentTypeID'); ga('send', 'pageview');
If you use GTM put it in dataLayer variable and take care of it in GTM
@Html.Raw("dataLayer = [{ 'dimension1': '" + Model.Layout.CurrentContent.ContentGuid + "', 'dimension2': '" + Model.Layout.CurrentContent.ContentTypeID + "'}];")
NOW get this in production, else no result will show up in next steps.
You should now see in the request to GA, that two new parameters is sent as a querystring (cd1 and cd2)
//www.google-analytics.com/collect?…&cd1=3df4975f-928e-41cc-bb66-ed54f71c36ad&125&cd2=125
Enable the API in Google APIs and Services
- Go to https://console.developers.google.com
- Create a project
- Click Enable Apis and services
- Search for Google Analytics Reporting API
- Add the API, and ENABLE IT
Register a service account and download a service account key
https://console.developers.google.com/apis/credentials
- Click Credentials – Create Credentials à service account key
- Select Any Role – viewer-permissions
- Select Json (P12 is not working in Azure)
- Download the json key file
- Beware of the Service Email – will be used next
Register the service account in Google Analytics User management
https://analytics.google.com/analytics/web/
- In Google Analytics – go to your account
- Admin / User management
- Add new user, the service email is this format: myservicename@myprojectname.iam.gserviceaccount.com and you can find it in the json file
- Give Read and Analyze permission
Configure the GoogleAnalyticsService
Code V4: https://gist.github.com/LucGosso/00ac7a2ece9c135ebf83ca897a0a8ba9
If you somehow are using V3, use this code: https://gist.github.com/LucGosso/d74e8299bbcc2084087f81c198c391d6 (the difference between 3 and 4 is a larger API and that new feature are only added to V4. V3 is still 2018 maintained)
From nuget.org, install-package Google.Apis.AnalyticsReporting.v4 in project
In web.config, add two appsettings
<add key="GoogleAnalyticsProfileId" value="15555555" /> <add key="GoogleAnalyticsKeyFilePath" value="~/configs/myproject-e18e6ff7c713.json" />
GoogleAnalyticsProfileId is found in GA admin/Account/propery/view/View Settings/view ID (https://analytics.google.com/analytics/web/)
Using the GoogleAnalyticsService.cs
There is a Linq extension OrderByMostPopular()
Example of use
List<PageData> pages = GetBlogItems().OrderByDescending(x => x.StartPublish).Take(20).OrderByMostPopular().ToList<PageData>()
This example returns 20 lastest published blog items in Most Popular order.
- NOTE If no data is found I GA it will filter away these pages
- NOTE if you have no data in GA, it will return empty list.
Alternatives
In GoogleAnalyticsService.cs there is two public methods GetItemViewsfromAnalytics and FilterItemsSortByMostPopular
public Dictionary<Guid, PageItemViews> GetItemViewsfromAnalytics(int typeid = 0, bool useCache = true)
The method is getting data from GA, you may get data for a specific typeid or all pageviews
public IEnumerable<PageData> FilterItemsSortByMostPopular(Dictionary<Guid, PageItemViews> pagesViews, IEnumerable<PageData> articles, string firstSegmentUrl = null)
Parameter pageview – the data from GA (from GetItemViewsfromAnalytics)
Parameter articles – is the content you want to sort
Parameter firstSegmentUrl – optional – if you want to filter the from first segment eg could be “en” for English pages or “products” if you’d have content under “example.com/products/someproduct/article/”Example of use
List<PageData> pages = GetBlogItems().OrderByDescending(x => x.StartPublish).Take(20).ToList<PageData>()
googleAnalyticsService.FilterItemsSortByMostPopular(googleAnalyticsService.GetItemViewsfromAnalytics(), pages).ToList(); //returning pages sorted by most viewed
- NOTE If no data is found I GA it will filter away these pages
- NOTE if you have no data in GA, it will return empty list.
Updating the cache with a scheduled job
By using googleAnalyticsService.GetItemViewsfromAnalytics(typeid: 0, useCache: false); you do update the cache and use some fresh data from GA.
SEO Terms
- Sort by number of views
- Most Popular listing from Analytics
- Trending and Hot topics based on Google Analytics
- Apis.Requests.RequestError Access Not Configured. Google Analytics API has not been used in project * before or it is disabled. (Don’t forget to enable the API)
- Google Analytics Api example
Resources
- https://gist.github.com/LucGosso/00ac7a2ece9c135ebf83ca897a0a8ba9
- https://developers.google.com/analytics/devguides/reporting/core/v4/
- https://console.developers.google.com/
About the author
Luc Gosso
– Independent Senior Web Developer
working with Azure and EpiserverTwitter: @LucGosso
LinkedIn: linkedin.com/in/luc-gosso/
Github: github.com/lucgosso