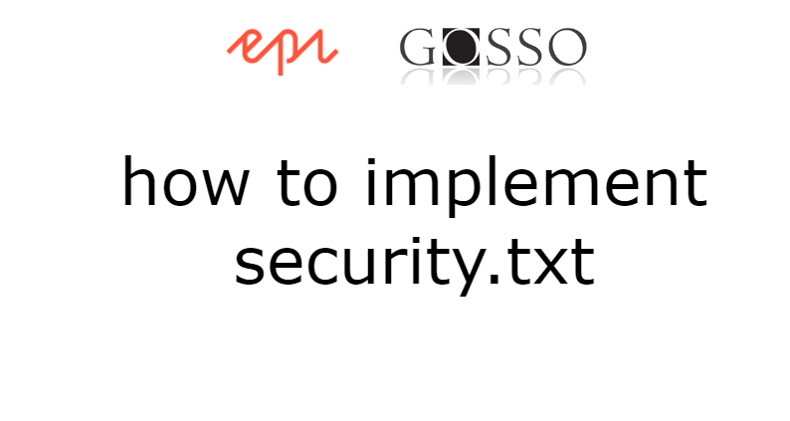
How to implement security.txt under .well-known in IIS
Focused on security? aren't we all? security.txt is a file that inform where you can report vulnerabilities on your site.
Published 14th of June 2018
When security risks in web services are discovered by independent security researchers who understand the severity of the risk, they often lack the channels to disclose them properly. As a result, security issues may be left unreported. Security.txt defines a standard to help organizations describe the process for security researchers to disclose security vulnerabilities securely. *
Security.txt is a proposed standard which allows website owners to define how to report security vulnerabilities. Security.txt is the equivalent of robots.txt
, but for security issues.
.well-known directory under IIS
Security.txt is supposed to be under path /.well-known/security.txt
It is acceptable to have it in the root dir but it should redirect to well-known path.
Folder paths with “.” (dot) is not allowed in IIS. The reasoning behind it is that it might give away sensitive information, like a .git or .svn directory (which probably shouldn’t even be on your webserver in the first place).
ONE way around this is to make a handler:
namespace Gosso.Website { using System.Web; public class SecurityTxtHandler : IHttpHandler { public bool IsReusable => true; public void ProcessRequest(HttpContext context) { if (context.Request.Path.ToLower() == "/security.txt") //redirect to /.well-known/ context.Response.RedirectPermanent("/.well-known/security.txt", true); context.Response.ContentType = "text/plain"; context.Response.WriteFile("~/security.txt"); //have a file in root dir } } }
Register the handler in web.config:
<system.webServer> <handlers> <add name="securitytxt1" path="*known/security.txt" verb="GET" type="Gosso.Website.SecurityTxtHandler" /> <add name="securitytxt2" path="/security.txt" verb="GET" type="Gosso.Website.SecurityTxtHandler" />
Simple implementation in Episerver
Make it editable from the CMS. Things change and editors may want to change content without changing a file on the server.
Just make a textarea property on your startpage called “SecurityText” and then use this handler:
namespace Gosso.Website { using System.Web; public class SecurityTxtHandler : IHttpHandler { public bool IsReusable => true; public void ProcessRequest(HttpContext context) { if (context.Request.Path.ToLower() == "/security.txt") context.Response.RedirectPermanent("/.well-known/security.txt", true); context.Response.ContentType = "text/plain"; var startpage = ServiceLocator.Current.GetInstance<IContentLoader>().Get<PageData>(ContentReference.StartPage); if (startpage.Property.TryGetPropertyValue("SecurityText", out string txt)) { context.Response.Write(txt); } else { context.Response.WriteFile("~/security.txt"); } } } }
Code is multisite proof
Content of the security.txt
Minimum example:
contact: https://www.example.com/contact/
With encryption key
contact: security@example.com encryption: https://example.com/pgp-key.txt
Parameters
Contact can be an email or a link to a form
Encryption is public key that can be used to encrypt the report
Policy is link to your security policy and/or disclosure policy
Acknowledgment is for owners to give kudos to security reporters
Hiring is for security professional hiring openings
Signature is for path to .sig file, so you can sign the security.txt
file
Who uses security.txt?
Contact: https://www.facebook.com/whitehat/report/ Acknowledgments: https://www.facebook.com/whitehat/thanks/ Policy: https://www.facebook.com/whitehat/info/ Hiring: https://www.facebook.com/careers/teams/security/
Contact: https://g.co/vulnz Contact: mailto:security@google.com Encryption: https://services.google.com/corporate/publickey.txt Acknowledgement: https://bughunter.withgoogle.com/ Policy: https://g.co/vrp Hiring: https://g.co/SecurityPrivacyEngJobs
# Dropbox uses HackerOne for responsible disclosure. # Please report abusive content (including malware, spam, etc) to abuse@dropbox.com. Contact: https://hackerone.com/dropbox/ Acknowledgements: https://hackerone.com/dropbox/thanks Policy: https://hackerone.com/dropbox/ Hiring: https://www.dropbox.com/jobs/listing/815756
Resources
- https://securitytxt.org/
- * https://github.com/securitytxt/security-txt
- https://ma.ttias.be/well-known-directory-webservers-aka-rfc-5785/
- https://www.michalspacek.com/what-is-security.txt-and-why-you-should-have-one
- https://twitter.com/troyhunt/status/962063783223902208
- https://blog.yeswehack.com/2018/02/06/interview-of-edoverflow-bug-hunter-mastermind-of-security-txt/
SEO terms
- What is security.txt
- How implement security.txt on IIS windows server
- How to make a .well-known directory in IIS
- examples of security.txt
About the author
Luc Gosso
– Independent Senior Web Developer
working with Azure and Episerver
Twitter: @LucGosso
LinkedIn: www.linkedin.com/in/luc-gosso/
Github: http://github.com/lucgosso